1、使用 os/exec 中的exec.Command方法来实现
package main
import (
"fmt"
"os/exec"
)
func main(){
cmd := exec.Command("ipconfig", "/all")
output, err := cmd.CombinedOutput()
if err != nil {
fmt.Println(fmt.Sprint(err) + ":" + string(output))
}
fmt.Println(string(output))
}
运行
go run .
结果... 乱码
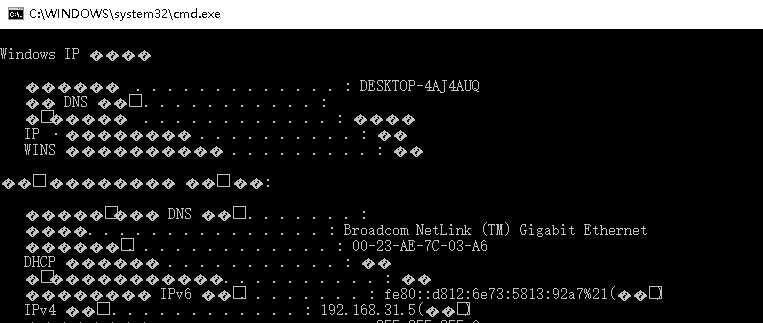
2、解决 Go exec.Command 乱码
这个问题主要应该还是在Windows下出现,Linux下工具没有提示中文信息,所以没有遇到这个问题
如果命令执行结果输出的是英文提示信息,在 命令行提示下 输入
chcp 65001
应该就可以正常了。
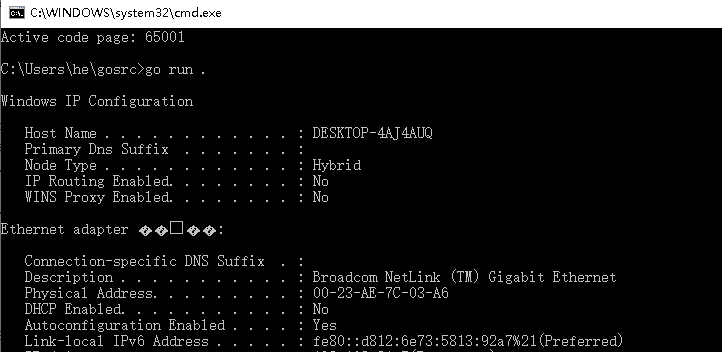
3、解决 Go exec.Command 中文乱码
中文乱码的问题没什么好方案了,只能老老实实转码了。
需要引入一个新的mod
go get -v golang.org/x/text/encoding/simplifiedchinese
提示这个才叫正确
go: downloading golang.org/x/text v0.3.6
go: found golang.org/x/text/encoding/simplifiedchinese in golang.org/x/text v0.3.6
golang.org/x/text/encoding/internal/identifier
golang.org/x/text/transform
golang.org/x/text/encoding
golang.org/x/text/encoding/internal
golang.org/x/text/encoding/simplifiedchinese
如果提示类似错误信息
go get golang.org/x/text/encoding/simplifiedchinese: module golang.org/x/text/encoding/simplifiedchinese: Get "https://proxy.golang.org/golang.org/x/text/encoding/simplifiedchinese/@v/list": dial tcp 172.217.160.81:443: connectex: A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond.
就加个http_proxy吧
set http_proxy=http://localhost:1080
完整的代码
package main
import (
"fmt"
"os/exec"
"golang.org/x/text/encoding/simplifiedchinese"
)
type Charset string
const (
UTF8 = Charset("UTF-8")
GB18030 = Charset("GB18030")
)
func main(){
cmd := exec.Command("ipconfig", "/all")
output, err := cmd.CombinedOutput()
if err != nil {
fmt.Println(fmt.Sprint(err) + ":" + string(output))
}
cmdRe:=ConvertByte2String([]byte(string(output)),"GB18030")
fmt.Println(cmdRe)
}
func ConvertByte2String(byte []byte, charset Charset) string {
var str string
switch charset {
case GB18030:
var decodeBytes,_=simplifiedchinese.GB18030.NewDecoder().Bytes(byte)
str= string(decodeBytes)
case UTF8:
fallthrough
default:
str = string(byte)
}
return str
}
运行结果:
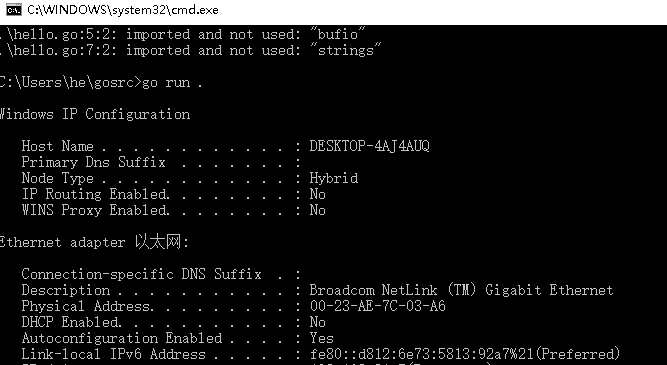